Spring Security - 405 Request Method 'POST' Not Supported. Ask Question Asked 3 years, 8 months ago. Request method 'POST' not supported. Spring version: 4.0.2. In the last post we tried securing our Spring MVC app using spring security Spring Boot Security Login Example.We protected our app against CSRF attack too. Today we will see how to secure REST Api using Basic Authentication with Spring security features.Here we will be using Spring boot to avoid basic configurations and complete java config.We will try to perform simple CRUD operation using. Spring Security 3.2+ provides support for setting X-Frame-Options on every response. By default the Spring Security Java config sets it to DENY. In 3.2 the Spring Security XML namespace does not set that header by default but may be configured to do so, and in the future it may set it by default. See Section 7.1. When using /login, I get an 'HTTP 404 Page Not Found' exception, and when I use /jsecuritycheck action, I get a 'HTTP Status 405 – Request method ‘POST' not supported' exception.
If you've never heard of JWTs (JSON Web Tokens), well, you don't work in tech, or you've purposely unplugged your computer from the Internet. JWTs are frequently used in OAuth2 as access and refresh tokens as well as a variety of other applications.
JWTs can be used wherever you need a stand-in to represent a 'user' of some kind (in quotes, because the user could be another microservice). And, they're used where you want to carry additional information beyond the value of the token itself and have that information cryptographically verifiable as security against corruption or tampering.
For more information on the background and structure of JWTs, here's the IETF specification
The code that backs this post can be found on GitHub.
Spring Security & CSRF Protection
CSRF (Cross Site Request Forgery) is a technique in which an attacker attempts to trick you into performing an action using an existing session of a different website.
Spring Security when combined with Thymeleaf templates, automatically inserts a token into all web forms as a hidden field. This token must be present on form submission, or a Java Exception is thrown. This mitigates the risk of CSRF as an external site (an attacker) would not be able to reproduce this token.
For this sample project, the following dependencies are all that's required to get Spring Boot, Spring Security, and Thymeleaf:
2 4 6 8 | <html lang='en'xmlns:th='http://www.thymeleaf.org'> <form method='post'th:action='@{/jwt-csrf-form}'> <input type='submit'class='btn btn-primary'value='Click Me!'/> </body> |
Notice the xmlns:th
attribute in the html
tag as well as th:action
attribute of the form
tag. It's these attributes that triggers Spring Security to inject the CSRF protection token into the form. Here's what that looks like:
2 | <input type='hidden'name='_csrf'value='72501b07-8205-491d-ba95-ebab5cf450de'/> |
This is what's called a 'dumb' token. Spring Security keeps a record of this token, usually in the user's session. When the form is submitted, it compares the value of the token to what Spring Security has on record. If the token is not present or is not the right value, an Exception is thrown.
We can improve on this using a JWT in the following ways:
- Ensure that a given token can only be used once by using a nonce cache
- Set a short expiration time for added security
- Verify that the token hasn't been tampered with using cryptographic signatures
Switching to JWTs for CSRF Protection
The JJWT (Java JWT) library is the premier open-source Java library for working with JSON Web Tokens. It's clean design including a fluent interface has led to over 1,000 stars on Github.
We can easily add the JJWT library to our project by dropping in the following dependency:

2 4 6 8 10 12 14 16 18 20 | publicCsrfToken generateToken(HttpServletRequest request){ Stringid=UUID.randomUUID().toString().replace('-','); Date exp=newDate(now.getTime()+(1000*30));// 30 seconds .setId(id) .setNotBefore(now) .signWith(SignatureAlgorithm.HS256,secret) returnnewDefaultCsrfToken('X-CSRF-TOKEN','_csrf',token); |
Here we see the JJWT fluent interface in action. We chain all the claims settings together and call the compact
terminator method to give us the final JWT string. Most importantly, this JWT will expire after 30 seconds.
The saveToken
and loadToken
methods do just what they say. In this example, they are saving the token to and loading the token from the user's session.
CSRF Token Validator
Spring Security will already do the 'dumb' part of the CSRF check and verify that the string it has stored matches the string that's passed in exactly. In addition, we want to leverage the the information encoded in the JWT. This is implemented as a filter.
Here's the core of the JwtCsrfValidatorFilter
:
2 4 6 8 10 12 | // CsrfFilter already made sure the token matched. Here, we'll make sure it's not expired Jwts.parser() .setSigningKeyResolver(secretService.getSigningKeyResolver()) }catch(JwtExceptione){ // most likely an ExpiredJwtException, but this will handle any response.setStatus(HttpServletResponse.SC_BAD_REQUEST); RequestDispatcher dispatcher=request.getRequestDispatcher('expired-jwt'); } |
If the JWT is parseable, processing will continue. As you can see in the catch
block, if parsing the JWT fails for any reason, we forward the request to an error page.
Spring Security Configuration
The Spring Security configuration ties it all together by registering our CSRF Token Repository with Spring Security. Here's the configure
method:
2 4 6 8 10 12 | protectedvoidconfigure(HttpSecurity http)throwsException{ .addFilterAfter(newJwtCsrfValidatorFilter(),CsrfFilter.class) .csrfTokenRepository(jwtCsrfTokenRepository) .and() .antMatchers('/**') } |
Line 3 adds our validator filter after the default Spring Security CSRF Filter.
Line 6 tells Spring Security to use our JWT CSRF Token Repository instead of the default one. Battlefield 1 free pc game.
JWT CSRF Protection in Action
To run the sample app, clone the GitHub repo and execute:
@ComponentScan(basePackages = { 'org.myakasha.crm','org.myakasha.crm.controller','org.myakasha.crm.model'})
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter{
@Autowired
DataSource dataSource;
@Autowired
public void registerAuthentication(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication().dataSource(dataSource)
.usersByUsernameQuery('select username,password, enabled from users where username=?')
.authoritiesByUsernameQuery('select username, role from user_roles where username=?');
}
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring().antMatchers('/resources/**');
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers('/admin/**').access('hasRole('ROLE_ADMIN')')
.and() Crack teamviewer 10 download.
.formLogin().loginPage('/login').failureUrl('/login?error').usernameParameter('username').passwordParameter('password')
.and()
.logout().logoutSuccessUrl('/login?logout')
.and()
.exceptionHandling().accessDeniedPage('/403')
.and()
.csrf();
}
}
SecurityController.java @Controller
public class SecurityController {
@RequestMapping(value = { '/welcome**' }, method = RequestMethod.GET)
public ModelAndView defaultPage() {
ModelAndView model = new ModelAndView();
model.addObject('title', 'Spring Security + Hibernate Example');
model.addObject('message', 'This is default page!');
model.setViewName('hello');
return model;
}
@RequestMapping(value = '/admin**', method = RequestMethod.GET)
public ModelAndView adminPage() {
ModelAndView model = new ModelAndView();
model.addObject('title', 'Spring Security + Hibernate Example');
model.addObject('message', 'This page is for ROLE_ADMIN only!');
model.setViewName('admin');
return model;
}
@RequestMapping(value = '/login', method = {RequestMethod.GET} )
public ModelAndView login(@RequestParam(value = 'error', required = false) String error,

@RequestParam(value = 'logout', required = false) String logout, HttpServletRequest request) {
ModelAndView model = new ModelAndView();
Request Method 'post' Not Supported Spring Security
if (error != null) {
model.addObject('error', getErrorMessage(request, 'SPRING_SECURITY_LAST_EXCEPTION'));
}
if (logout != null) {
model.addObject('msg', 'You've been logged out successfully.');
}
model.setViewName('login');
return model;
}
// customize the error message
private String getErrorMessage(HttpServletRequest request, String key) {
Exception exception = (Exception) request.getSession().getAttribute(key);
String error = ';
if (exception instanceof BadCredentialsException) {
error = 'Invalid username and password!';
} else if (exception instanceof LockedException) {
error = exception.getMessage();
} else {
error = 'Invalid username and password!';
}
return error;
}
// for 403 access denied page
@RequestMapping(value = '/403', method = RequestMethod.GET)
public ModelAndView accesssDenied() {
ModelAndView model = new ModelAndView();
// check if user is login
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (!(auth instanceof AnonymousAuthenticationToken)) {
UserDetails userDetail = (UserDetails) auth.getPrincipal();
System.out.println(userDetail);
model.addObject('username', userDetail.getUsername());
}
model.setViewName('403');
return model;
}
}
WebConfig.java @EnableAutoConfiguration
@EnableWebMvc
@ComponentScan(basePackages = {'org.myakasha.crm','org.myakasha.crm.controller','org.myakasha.crm.model'})
public class WebConfig extends WebMvcConfigurerAdapter{
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
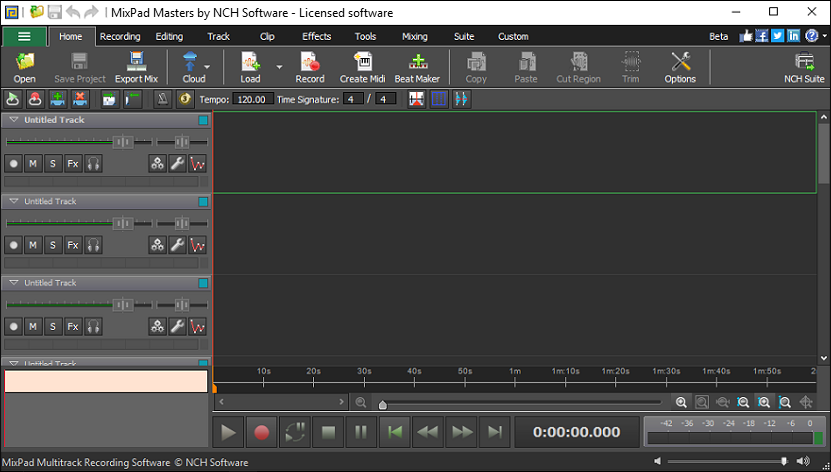
configurer.enable();
}
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler('/resources/**').addResourceLocations('/resources/');
}
/**
* This function to replace servlet-content.xml
* Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory
**/
@Bean
public InternalResourceViewResolver getInternalResourceViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver .setPrefix('/WEB-INF/views/');
viewResolver .setSuffix('.jsp');
return viewResolver ;
}
@Bean
public MessageSource messageSource() {
ReloadableResourceBundleMessageSource messageSource = new ReloadableResourceBundleMessageSource();
messageSource.setBasenames('classpath:message');
messageSource.setUseCodeAsDefaultMessage(true);
messageSource.setDefaultEncoding('UTF-8');
return messageSource;

}
}
PersistenceConfig.java @Configuration
@EnableTransactionManagement
@PropertySource({ 'classpath:persistence-mysql.properties' })
@ComponentScan({ 'org.myakasha.crm' })
public class PersistenceConfig {
@Autowired
private Environment env;
public PersistenceConfig() {
super();
}
@Bean
public LocalSessionFactoryBean sessionFactory() {
final LocalSessionFactoryBean sessionFactory = new LocalSessionFactoryBean();
sessionFactory.setDataSource(restDataSource());
sessionFactory.setPackagesToScan(new String[] { 'org.myakasha.crm.model' });
sessionFactory.setHibernateProperties(hibernateProperties());
return sessionFactory;
}
@Bean
public DataSource restDataSource() {
final BasicDataSource dataSource = new BasicDataSource();
dataSource.setDriverClassName(Preconditions.checkNotNull(env.getProperty('jdbc.driverClassName')));
dataSource.setUrl(Preconditions.checkNotNull(env.getProperty('jdbc.url')));
dataSource.setUsername(Preconditions.checkNotNull(env.getProperty('jdbc.user')));
dataSource.setPassword(Preconditions.checkNotNull(env.getProperty('jdbc.pass')));
return dataSource;
}
@Bean
@Autowired
public HibernateTransactionManager transactionManager(final SessionFactory sessionFactory) {
final HibernateTransactionManager txManager = new HibernateTransactionManager();
txManager.setSessionFactory(sessionFactory);
return txManager;
}
@Bean
public PersistenceExceptionTranslationPostProcessor exceptionTranslation() {
return new PersistenceExceptionTranslationPostProcessor();
}
final Properties hibernateProperties() {
final Properties hibernateProperties = new Properties();
hibernateProperties.setProperty('hibernate.hbm2ddl.auto', env.getProperty('hibernate.hbm2ddl.auto'));
hibernateProperties.setProperty('hibernate.dialect', env.getProperty('hibernate.dialect'));
2 4 6 8 10 12 14 16 18 20 | publicCsrfToken generateToken(HttpServletRequest request){ Stringid=UUID.randomUUID().toString().replace('-','); Date exp=newDate(now.getTime()+(1000*30));// 30 seconds .setId(id) .setNotBefore(now) .signWith(SignatureAlgorithm.HS256,secret) returnnewDefaultCsrfToken('X-CSRF-TOKEN','_csrf',token); |
Here we see the JJWT fluent interface in action. We chain all the claims settings together and call the compact
terminator method to give us the final JWT string. Most importantly, this JWT will expire after 30 seconds.
The saveToken
and loadToken
methods do just what they say. In this example, they are saving the token to and loading the token from the user's session.
CSRF Token Validator
Spring Security will already do the 'dumb' part of the CSRF check and verify that the string it has stored matches the string that's passed in exactly. In addition, we want to leverage the the information encoded in the JWT. This is implemented as a filter.
Here's the core of the JwtCsrfValidatorFilter
:
2 4 6 8 10 12 | // CsrfFilter already made sure the token matched. Here, we'll make sure it's not expired Jwts.parser() .setSigningKeyResolver(secretService.getSigningKeyResolver()) }catch(JwtExceptione){ // most likely an ExpiredJwtException, but this will handle any response.setStatus(HttpServletResponse.SC_BAD_REQUEST); RequestDispatcher dispatcher=request.getRequestDispatcher('expired-jwt'); } |
If the JWT is parseable, processing will continue. As you can see in the catch
block, if parsing the JWT fails for any reason, we forward the request to an error page.
Spring Security Configuration
The Spring Security configuration ties it all together by registering our CSRF Token Repository with Spring Security. Here's the configure
method:
2 4 6 8 10 12 | protectedvoidconfigure(HttpSecurity http)throwsException{ .addFilterAfter(newJwtCsrfValidatorFilter(),CsrfFilter.class) .csrfTokenRepository(jwtCsrfTokenRepository) .and() .antMatchers('/**') } |
Line 3 adds our validator filter after the default Spring Security CSRF Filter.
Line 6 tells Spring Security to use our JWT CSRF Token Repository instead of the default one. Battlefield 1 free pc game.
JWT CSRF Protection in Action
To run the sample app, clone the GitHub repo and execute:
@ComponentScan(basePackages = { 'org.myakasha.crm','org.myakasha.crm.controller','org.myakasha.crm.model'})
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter{
@Autowired
DataSource dataSource;
@Autowired
public void registerAuthentication(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication().dataSource(dataSource)
.usersByUsernameQuery('select username,password, enabled from users where username=?')
.authoritiesByUsernameQuery('select username, role from user_roles where username=?');
}
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring().antMatchers('/resources/**');
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers('/admin/**').access('hasRole('ROLE_ADMIN')')
.and() Crack teamviewer 10 download.
.formLogin().loginPage('/login').failureUrl('/login?error').usernameParameter('username').passwordParameter('password')
.and()
.logout().logoutSuccessUrl('/login?logout')
.and()
.exceptionHandling().accessDeniedPage('/403')
.and()
.csrf();
}
}
SecurityController.java @Controller
public class SecurityController {
@RequestMapping(value = { '/welcome**' }, method = RequestMethod.GET)
public ModelAndView defaultPage() {
ModelAndView model = new ModelAndView();
model.addObject('title', 'Spring Security + Hibernate Example');
model.addObject('message', 'This is default page!');
model.setViewName('hello');
return model;
}
@RequestMapping(value = '/admin**', method = RequestMethod.GET)
public ModelAndView adminPage() {
ModelAndView model = new ModelAndView();
model.addObject('title', 'Spring Security + Hibernate Example');
model.addObject('message', 'This page is for ROLE_ADMIN only!');
model.setViewName('admin');
return model;
}
@RequestMapping(value = '/login', method = {RequestMethod.GET} )
public ModelAndView login(@RequestParam(value = 'error', required = false) String error,
@RequestParam(value = 'logout', required = false) String logout, HttpServletRequest request) {
ModelAndView model = new ModelAndView();
Request Method 'post' Not Supported Spring Security
if (error != null) {
model.addObject('error', getErrorMessage(request, 'SPRING_SECURITY_LAST_EXCEPTION'));
}
if (logout != null) {
model.addObject('msg', 'You've been logged out successfully.');
}
model.setViewName('login');
return model;
}
// customize the error message
private String getErrorMessage(HttpServletRequest request, String key) {
Exception exception = (Exception) request.getSession().getAttribute(key);
String error = ';
if (exception instanceof BadCredentialsException) {
error = 'Invalid username and password!';
} else if (exception instanceof LockedException) {
error = exception.getMessage();
} else {
error = 'Invalid username and password!';
}
return error;
}
// for 403 access denied page
@RequestMapping(value = '/403', method = RequestMethod.GET)
public ModelAndView accesssDenied() {
ModelAndView model = new ModelAndView();
// check if user is login
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (!(auth instanceof AnonymousAuthenticationToken)) {
UserDetails userDetail = (UserDetails) auth.getPrincipal();
System.out.println(userDetail);
model.addObject('username', userDetail.getUsername());
}
model.setViewName('403');
return model;
}
}
WebConfig.java @EnableAutoConfiguration
@EnableWebMvc
@ComponentScan(basePackages = {'org.myakasha.crm','org.myakasha.crm.controller','org.myakasha.crm.model'})
public class WebConfig extends WebMvcConfigurerAdapter{
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
configurer.enable();
}
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler('/resources/**').addResourceLocations('/resources/');
}
/**
* This function to replace servlet-content.xml
* Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory
**/
@Bean
public InternalResourceViewResolver getInternalResourceViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver .setPrefix('/WEB-INF/views/');
viewResolver .setSuffix('.jsp');
return viewResolver ;
}
@Bean
public MessageSource messageSource() {
ReloadableResourceBundleMessageSource messageSource = new ReloadableResourceBundleMessageSource();
messageSource.setBasenames('classpath:message');
messageSource.setUseCodeAsDefaultMessage(true);
messageSource.setDefaultEncoding('UTF-8');
return messageSource;
}
}
PersistenceConfig.java @Configuration
@EnableTransactionManagement
@PropertySource({ 'classpath:persistence-mysql.properties' })
@ComponentScan({ 'org.myakasha.crm' })
public class PersistenceConfig {
@Autowired
private Environment env;
public PersistenceConfig() {
super();
}
@Bean
public LocalSessionFactoryBean sessionFactory() {
final LocalSessionFactoryBean sessionFactory = new LocalSessionFactoryBean();
sessionFactory.setDataSource(restDataSource());
sessionFactory.setPackagesToScan(new String[] { 'org.myakasha.crm.model' });
sessionFactory.setHibernateProperties(hibernateProperties());
return sessionFactory;
}
@Bean
public DataSource restDataSource() {
final BasicDataSource dataSource = new BasicDataSource();
dataSource.setDriverClassName(Preconditions.checkNotNull(env.getProperty('jdbc.driverClassName')));
dataSource.setUrl(Preconditions.checkNotNull(env.getProperty('jdbc.url')));
dataSource.setUsername(Preconditions.checkNotNull(env.getProperty('jdbc.user')));
dataSource.setPassword(Preconditions.checkNotNull(env.getProperty('jdbc.pass')));
return dataSource;
}
@Bean
@Autowired
public HibernateTransactionManager transactionManager(final SessionFactory sessionFactory) {
final HibernateTransactionManager txManager = new HibernateTransactionManager();
txManager.setSessionFactory(sessionFactory);
return txManager;
}
@Bean
public PersistenceExceptionTranslationPostProcessor exceptionTranslation() {
return new PersistenceExceptionTranslationPostProcessor();
}
final Properties hibernateProperties() {
final Properties hibernateProperties = new Properties();
hibernateProperties.setProperty('hibernate.hbm2ddl.auto', env.getProperty('hibernate.hbm2ddl.auto'));
hibernateProperties.setProperty('hibernate.dialect', env.getProperty('hibernate.dialect'));
hibernateProperties.setProperty('hibernate.show_sql', 'true');
// hibernateProperties.setProperty('hibernate.format_sql', 'true');
// hibernateProperties.setProperty('hibernate.globally_quoted_identifiers', 'true');
return hibernateProperties;
}
}
spring spring-mvc spring-security spring-java-config
|
this question edited Oct 10 '14 at 15:25 asked Oct 10 '14 at 14:55 Eliz Liew 11 1 3 1 what request are you making that fails? all your annotations say method = RequestMethod.GET .. and the error suggests you're making a PosT – Birgit Martinelle Oct 10 '14 at 15:03 Trying to login from login form, it should be leading to login?error page. – Eliz Liew Oct 10 '14 at 15:21 how do you call that login? are you submitting a form with action='post'? – Birgit Martinelle Oct 10 '14 at 15:23 Yes you are right. – Eliz Liew Oct 10 '14 at 15:28 so change your @RequestMapping(value = '/login', method = {RequestMethod.GET} ) to @RequestMapping(value = '/login', method = {RequestMethod.POST} ) – Birgit Martinelle Oct 10 '14 at 15:30
up vote 1 down vote try to add login-processing-url to the SecurityConfig in XML it looks like this
login-page='/login'
login-processing-url='/login_process'
default-target-url='/home'
authentication-failure-url='/login?error'
username-parameter='username'
password-parameter='password' />
|
this answer answered Aug 6 '15 at 8:40 MAFH 11 1
|
this answer answered Jun 17 '16 at 13:33 Chathuranga Tennakoon 470 1 4 13
Request Method Post Not Supported
推荐:spring security
spring security http://ryanflyer.iteye.com/blog/973319 http://blog.csdn.net/k10509806/article/details/6369131
Request Method Post Not Supported Spring Security Entry
- 1使用Spring Security实现权限管理
- 2Spring Security 与 Oauth2 整合 步骤
- 3Spring 3之MVC & Security简单整合开发(二)
- 4spring session入门
- 5spring security控制权限的几种方法
- 1微信公众号文章采集,并发布到WordPress
- 2Spring Security
- 3spring security
Request Method 'post' Not Supported J_spring_security_check
最新文章
Spring Security Httprequestmethodnotsupportedexception Request Method 'post' Not Supported
- 1在阿里架构师眼中构建一个较为通用的业务技术架构就是如此简单
- 2Maven + Spring Boot + Mybatis 框架整合
- 3一分钟学会spring注解之@Lazy注解
- 4Spring Cloud构建分布式微服务云架构
- 5spring boot之从零开始开发自己的网站
- 6最近有人说我欺骗消费者,今天来一波视频分享